ブログ
これまでに経験してきたプロジェクトで気になる技術の情報を紹介していきます。
vuexの代わりにpiniaを使う
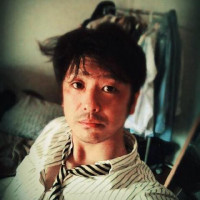
vuexの代わりにpiniaを使う
PiniaはVuexの後継となる状態管理(state management)ライブラリ。 現在は公式プロジェクトになっている。
Vuexでのmutations
を廃止し、 dispatch
や commit
などはなくストアの変更は単に定義したメソッドを呼び出すだけ。
同様にストアからの読み出しも単純にプロパティへのアクセスになる。
Composition APIでのComposition関数(composable)を使った簡潔な実装なのでVuexとくらべて学習コストが低くなる。
今までのvuexとの違いは以下のようなものがある
- TypeScript のフルサポート
- mutations の廃止
- ネストされたモジュールの廃止
- ネームスペースの廃止
- Code Spliting(コードを分割)
piniaのインストールと設定
npm install pinia
createPinia() を用いて Pinia をインストール
import { createApp } from "vue";
import { createPinia } from "pinia";
import App from "./App.vue";
createApp(App)
.use(createPinia())
.mount("#app");
storeの作成
基本的なストアを作成
defineStore
でストアを定義defineStore
の第一引数はアプリケーション内でユニークなキーdefineStore
の第二引数はストアの定義となるオブジェクトでstate
、getters
、actions
の3つのプロパティを指定- vuexと違いmutationsはない
// defineStoreをインポート
import {defineStore} from 'pinia'
// defineStore`でストアを定義
// 第一引数はアプリケーション内でユニークなキー
export const useTestStore = defineStore('test', {
// state -> ストアの初期状態を定義
state: () => ({
counter: 0,
}),
// getters -> 状態を取得するgetter(computedに相当)
getters: {
doubleCount: (state) => state.counter * 2,
},
// actions -> 状態を変更するaction(methodsに相当)
actions: {
increment()
{
this.count++
},
decrement()
{
this.counter--
},
}
})
使用方法
- storeToRefsでリアクティブを維持したまま分割代入可能
- toRefsだとreactivityが壊れるので注意
- actionはストアオブジェクトから直接呼び出せる
<template>
<div>
<p>counter: {{counter}}</p>
<p>doubleCount: {{doubleCount}}</p>
<button type="button" @click="increment">increment</button>
<!-- test.decrement()で直接呼び出しも可能 -->
<button type="button" @click="test.decrement()">decrement</button>
</div>
</template>
<script>
import {useTestStore} from '@/store/test'
import {storeToRefs} from 'pinia'
export default {
setup()
{
// ストアの呼び出し
const test = useTestStore()
// ストアプロパティの取得
// toRefsだとreactivityが壊れるので注意
const {counter, doubleCount} = storeToRefs(test)
// ストアアクションの取得
const increment = () => {
test.increment()
};
return {
test,
counter,
doubleCount,
increment,
}
},
}
</script>
vuexの代わりにpiniaを使う
vuexの代わりにpiniaを使う
2022-01-28 14:54:36
2022-01-28 15:14:05
コメントはありません。